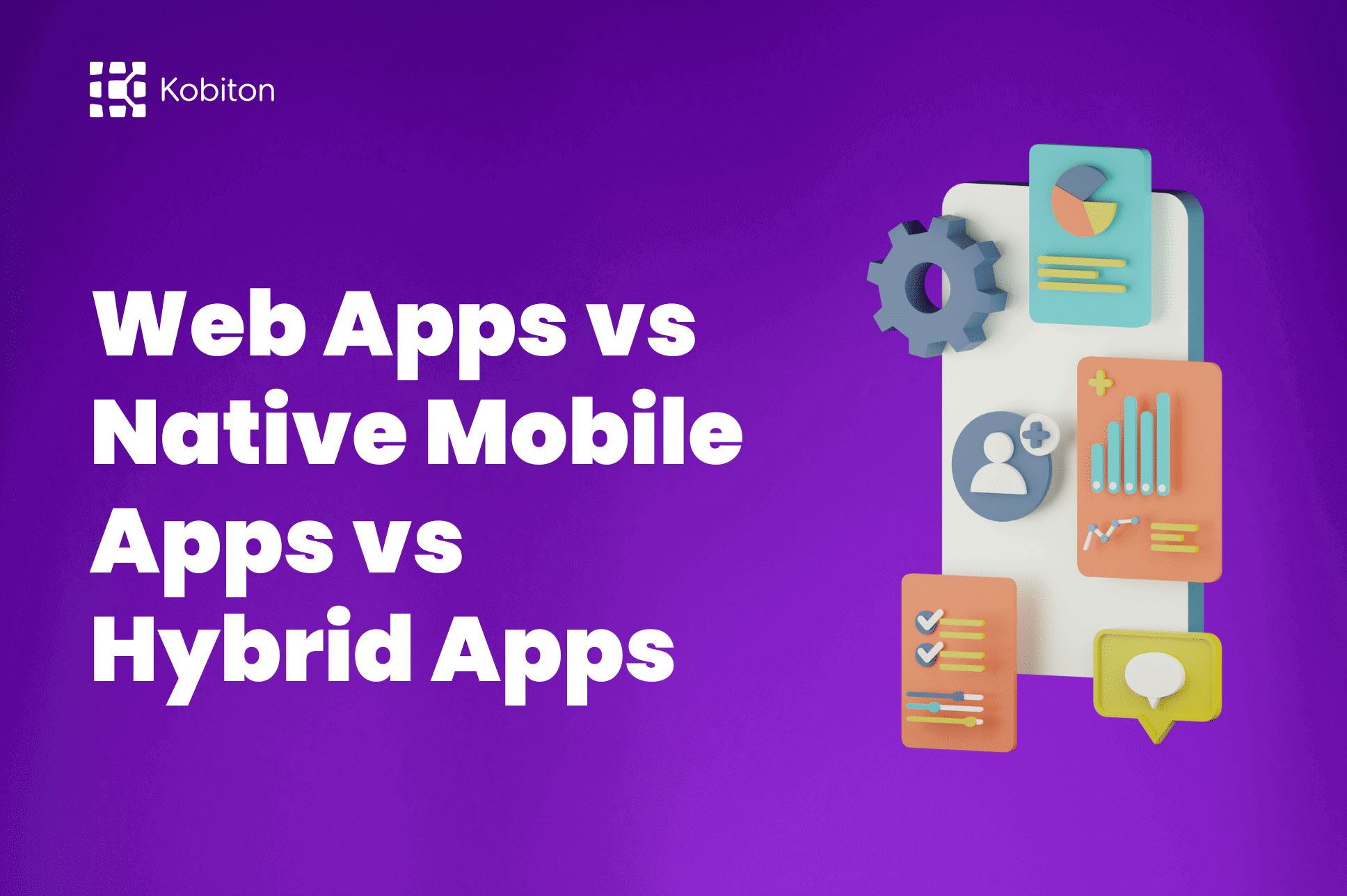
Web Apps vs Native Mobile Apps vs Hybrid Apps
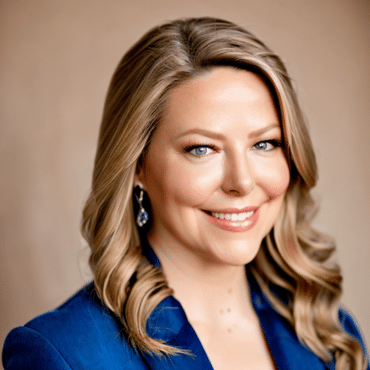
Cara Suarez
After developing a mobile app, it’s crucial to ensure it’s bug-free before launching. To develop a truly successful app, developers must always consider user experience. Achieving that means making sure your code works as intended.
This is where JUnit testing comes in. In this guide, we go over the basics of Junit, why it’s important, how to integrate it into development cycles, and the types of tests available. So if you’re keen to learn more about Junit testing for mobile app development, keep reading!
JUnit is a popular Java application testing framework. It enables developers to create automated tests that ensure their code works as expected. This makes it a simple and efficient way to test code, as it is easier to find bugs and ensure that code changes do not introduce new issues.
This testing app works by supplying a set of annotations and assertions for developers to use when defining test methods. A test runner can then run these test methods automatically, which compares the expected and actual results. This enables developers to quickly identify and fix issues with their code before they become more significant problems.
JUnit’s ability to assist developers in writing testable code is one of its primary advantages. Developers can create more reliable and maintainable software by designing code with testing in mind. JUnit can also be integrated with other development tools in order to provide a unified testing experience.
JUnit testing or Java unit testing is an essential tool for any Java developer looking to improve the quality of their code. It enables you to automate testing, identify bugs early and ensure that code changes don’t introduce new problems. It also enables developers to create more robust and dependable software, resulting in better user experiences and fewer headaches in the long run.
JUnit testing is an essential quality assurance method widely used in mobile app development. With this quick guide, you’ll learn how to use JUnit testing effectively to improve your mobile app’s functionality and user experience.
1. Set up the testing environment: To start, you need to set up the JUnit testing framework for your mobile app. This involves installing the JUnit library and integrating it with your development environment. Once done, you can create test cases to verify your app’s functionality.
2. Create test cases: JUnit testing involves creating test cases that simulate various scenarios and user actions in your mobile app. These test cases should cover all aspects of the app’s functionality, including UI, input validation, data storage, and network connectivity. By testing each component of your app thoroughly, you can identify and fix any bugs or errors before the app is released to users.
3. Carry out the tests: It is now time to run the test cases created. This process entails running the test cases and comparing the results to what was expected. Any differences between the two indicate that the app has a bug or error that needs to be fixed.
4. Examine the results: After running the tests, you can analyze the results to identify any issues that need to be addressed. You can quickly identify and resolve any bugs or errors that may affect the user experience by reviewing the results of the JUnit tests
When it comes to creating and running tests in JUnit, there are a few essential steps to follow. In this section, I’ll outline the process and provide examples of how tests are created and run in JUnit.
Create a Test Class
To begin, you’ll need to create a test class for your mobile app. This test class will contain various methods that test different aspects of your app’s functionality. Here is an example of a simple test class:
<code>
import org.junit.Test;
import static org.junit.Assert.*;
public class MyTest {
@Test
public void testAddition() {
int result = 1 + 2;
assertEquals(3, result);
}
}
</code>
In this example, the test class contains a single test method that checks if the addition of 1 and 2 is equal to 3. The ‘@Test annotation indicates that this is a test method.
Once you have created the test class, you can run it to verify that the tests pass. There are various ways to run the test class, depending on your IDE or build tool. Here is an example of how to run the test class using the command line:
<code>
javac -cp junit.jar MyTest.java
java -cp junit.jar:. org.junit.runner.JUnitCore MyTest
</code>
After running the test class, you can examine the results to identify any issues that require attention. JUnit includes a number of assertion methods for determining whether a test passes or fails. The assertEquals method is used in the preceding example test class to compare the expected result with the actual result of the addition.
How Assertions Work in JUnit Testing
In JUnit testing, assertions are crucial in ensuring that the code being tested functions as intended. Assertions are used to verify that a given condition is true during the execution of a test. In this section, I’ll explain how assertions work in Java Unit testing and provide examples to help illustrate their functionality.
When creating a test method, JUnit provides a range of assertion methods that can be used to verify expected outcomes. These methods include ‘assertTrue()’, ‘assertFalse()’, ‘assertEquals()’, ‘assertNotNull()’ and many others. Here’s a closer look at how some of these assertion methods work:
This method checks if a given boolean condition is true. If the condition is false, the test fails. Here’s an example:
<code>
@Test
public void testIsTrue() {
assertTrue(2 + 2 == 4);
}
</code>
This method checks if two values are equal to each other. If the values are not equal, the test fails. For example:
<code>
@Test
public void testAddition() {
int result = 1 + 2;
assertEquals(3, result);
}
</code>
This method checks if a given object is not null. If the object is null, the test fails. Here’s an example:
<code>
@Test
public void testObject() {
Object obj = new Object();
assertNotNull(obj);
}
</code>
When an assertion fails, the JUnit testing framework marks the test as failed and stops executing any additional assertions within the same test method. This ensures that any problems are identified and resolved as soon as possible.
Finally, assertions are an essential part of Java Unit testing because they help to ensure that the code works as expected. Developers can create comprehensive tests that thoroughly examine their code for bugs and errors by using assertion methods such as assertTrue(), assertEquals(), and assertNotNull().
Kobiton is a platform dedicated to creating better and more practical tests for development teams. With this service, JUnit testing can be executed without worrying about negatively impacting any code you’ve previously written.
Kobiton checks the code and applies appropriate tests for its integrity. Your team won’t need to rewrite tests or redo anything that has been previously added to your repository.
JUnit is the best java framework for building secure and useful tests. High-quality and reliable tests are often underestimated in the world of Java development, but with a valid sense of priority, it’s possible to do a fantastic job with it. Be sure to give the entire development team a heads-up before testing to avoid any interference.
You may also be interested in: