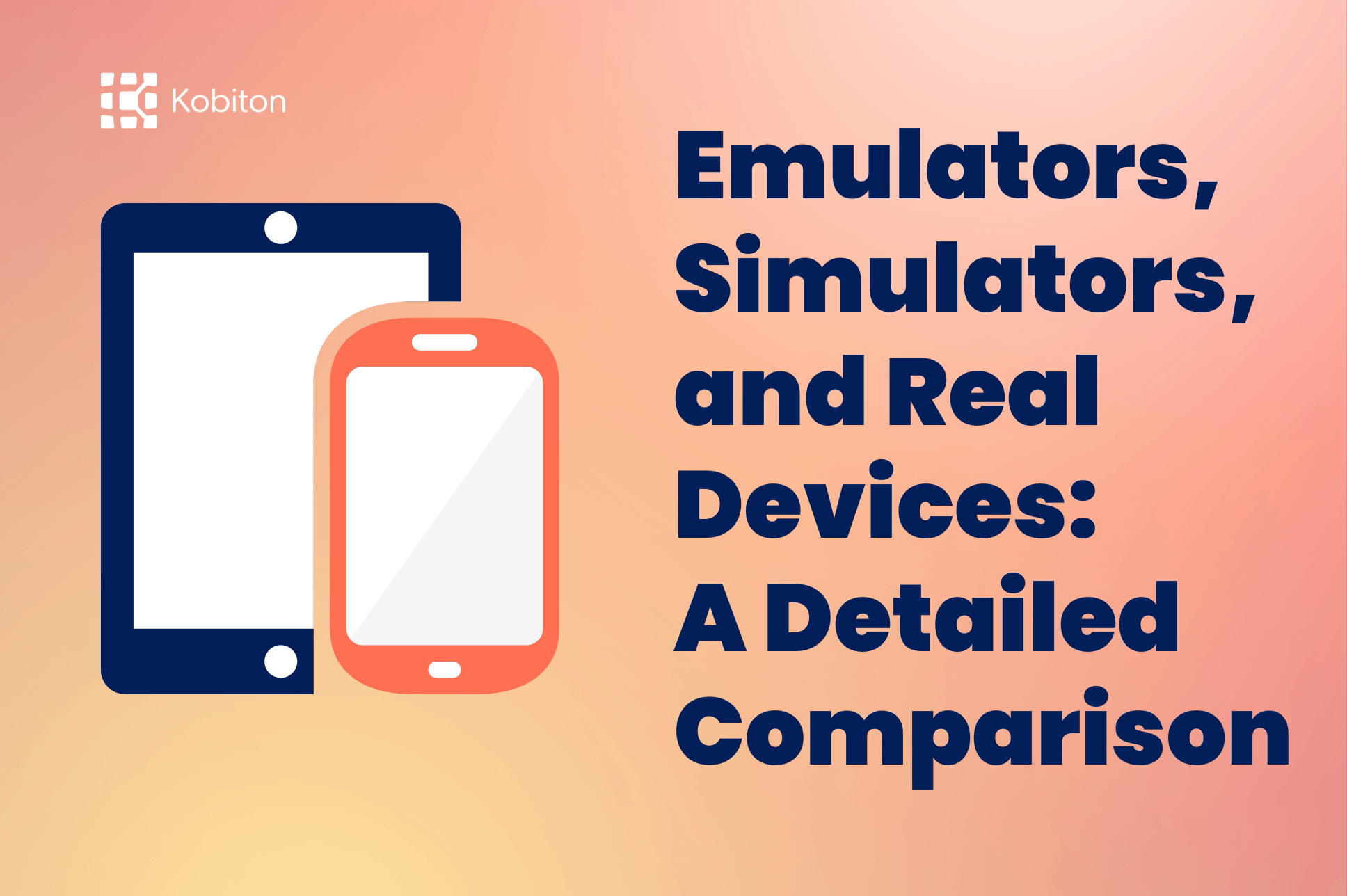
Emulators, Simulators, and Real Devices: A Detailed Comparison
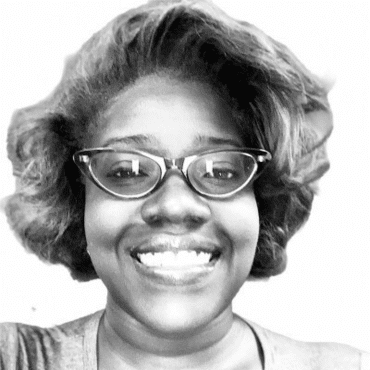
Brittney Lawrence
Kobiton is a cloud platform for executing automated and manual mobile and web tests. Kobiton supports running automated tests with Selenium WebDriver (for web applications) and Appium (for native and mobile web applications).
You can test your websites with your favorite language – no need to learn specific scripting languages or learn a new programming language. In addition, you also don’t need to install testing SDKs and even the Appium binding can be a pain point for test and dev team. When you run Appium in the cloud, there is no need to install Appium and set up your devices. You’ll have access to test on hundreds of browsers instantly.
Today, I’ll walk you through a basic example of how to use Appium / Selenium for mobile cross-browser testing using real Android and iOS devices and real web browsers on these platforms on Kobiton Cloud.
If you do not have a Kobiton account yet, go ahead to create a free trial account and sign in. It takes just a few moments.
After signing in, you should be on the Devices page. Hover over the device you want to test and select Show automation settings for Java language as below:
For Android device:
DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability("sessionName", "Automation test session on Android web"); capabilities.setCapability("sessionDescription", "This is example Android web testing"); capabilities.setCapability("deviceOrientation", "portrait"); capabilities.setCapability("captureScreenshots", true); capabilities.setCapability("browserName", "chrome"); capabilities.setCapability("deviceGroup", "KOBITON"); capabilities.setCapability("deviceName", "Galaxy A7"); capabilities.setCapability("platformVersion", "5.0.2"); capabilities.setCapability("platformName", "Android");
For iOS device:
DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability("sessionName", "Automation test session on iOS web"); capabilities.setCapability("sessionDescription", "This is example iOS web testing"); capabilities.setCapability("deviceOrientation", "portrait"); capabilities.setCapability("captureScreenshots", true); capabilities.setCapability("browserName", "safari"); capabilities.setCapability("deviceGroup", "KOBITON"); capabilities.setCapability("deviceName", "iPhone X"); capabilities.setCapability("platformVersion", "11.4.1"); capabilities.setCapability("platformName", "iOS");
Capture Screenshots
It allows skipping screenshots for each HTTP command or not. Depending on these screenshots, we can know the error or UI issue during running automation test. Please see the example:
capabilities.setCapability("captureScreenshots", true); // Or false
Portrait/Landscape
It’s possible to rotate the device before your test and you will have a different view of your website. Please see the example:
Rotate before test:
DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability("deviceOrientation", "portrait"); // Or "landscape"
After all these steps have been done, let’s look at how to use WebDriver to get the test running. First, you need to initiate the WebDriver. Note that if you are using client-side execution this really may take some time as the device will be prepared for your session.
String kobitonServerUrl = "https://joeybrown:3bfd8955-e252-4754-bffd-beb057b2b123@api.kobiton.com/wd/hub"; RemoteWebDriver driver = new RemoteWebDriver(new URL(kobitonServerUrl),capabilities);
This code example illustrates setting up a simple Java test to find the title of Appium page.
For Android device:
import org.openqa.selenium.remote.DesiredCapabilities; import org.openqa.selenium.remote.RemoteWebDriver; import java.net.URL; public class RemoteWebDriverAndroidTest { public static void main(String[] args) throws Exception { String kobitonServerUrl = "https://joeybrown:3bfd8955-e252-4754-bffd-beb057b2b123@api.kobiton.com/wd/hub"; DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability("sessionName", "Automation test session on Android web"); capabilities.setCapability("sessionDescription", "This is example Android web testing"); capabilities.setCapability("deviceOrientation", "portrait"); capabilities.setCapability("captureScreenshots", true); capabilities.setCapability("browserName", "chrome"); capabilities.setCapability("deviceGroup", "KOBITON"); capabilities.setCapability("deviceName", "Galaxy A7"); capabilities.setCapability("platformVersion", "5.0.2"); capabilities.setCapability("platformName", "Android"); RemoteWebDriver driver = new RemoteWebDriver(new URL(kobitonServerUrl), capabilities); /** * Prints Kobiton Session Id */ String kobitonSessionId = driver.getCapabilities().getCapability("kobitonSessionId").toString(); System.out.println("Your test session is: https://portal.kobiton.com/sessions/" + kobitonSessionId); driver.get("https://appium.io/"); /** * Goes to Appium page and prints URL & title */ System.out.println("Current URL is: " + driver.getCurrentUrl()); System.out.println("Title of page is: " + driver.getTitle()); driver.quit(); } }
For iOS device:
import org.openqa.selenium.remote.DesiredCapabilities; import org.openqa.selenium.remote.RemoteWebDriver; import java.net.URL; public class RemoteWebDriverIOSTest { public static void main(String[] args) throws Exception { String kobitonServerUrl = "https://joeybrown:3bfd8955-e252-4754-bffd-beb057b2b123@api.kobiton.com/wd/hub"; DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability("sessionName", "Automation test session on iOS web"); capabilities.setCapability("sessionDescription", "This is example iOS web testing"); capabilities.setCapability("deviceOrientation", "portrait"); capabilities.setCapability("captureScreenshots", true); capabilities.setCapability("browserName", "safari"); capabilities.setCapability("deviceGroup", "KOBITON"); capabilities.setCapability("deviceName", "iPhone X"); capabilities.setCapability("platformVersion", "11.4.1"); capabilities.setCapability("platformName", "iOS"); RemoteWebDriver driver = new RemoteWebDriver(new URL(kobitonServerUrl), capabilities); /** * Prints Kobiton Session Id */ String kobitonSessionId = driver.getCapabilities().getCapability("kobitonSessionId").toString(); System.out.println("Your test session is: https://portal.kobiton.com/sessions/" + kobitonSessionId); driver.get("https://appium.io/"); System.out.println("Current URL is: " + driver.getCurrentUrl()); System.out.println("Title of page is: " + driver.getTitle()); /** * Goes to Appium page and prints URL & title */ driver.quit(); } }
The result displays as below: