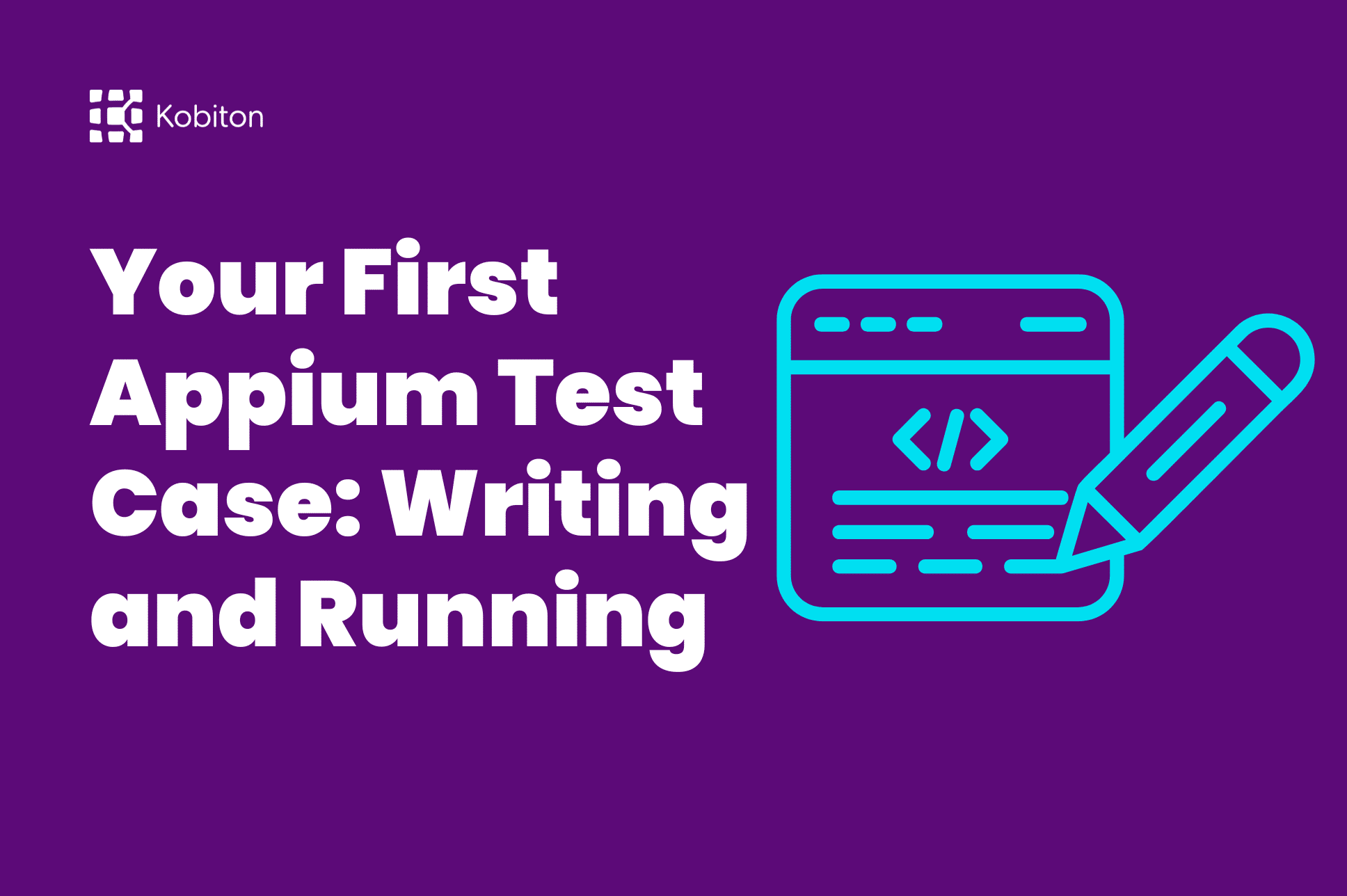
Your First Appium Test Case: Writing and Running
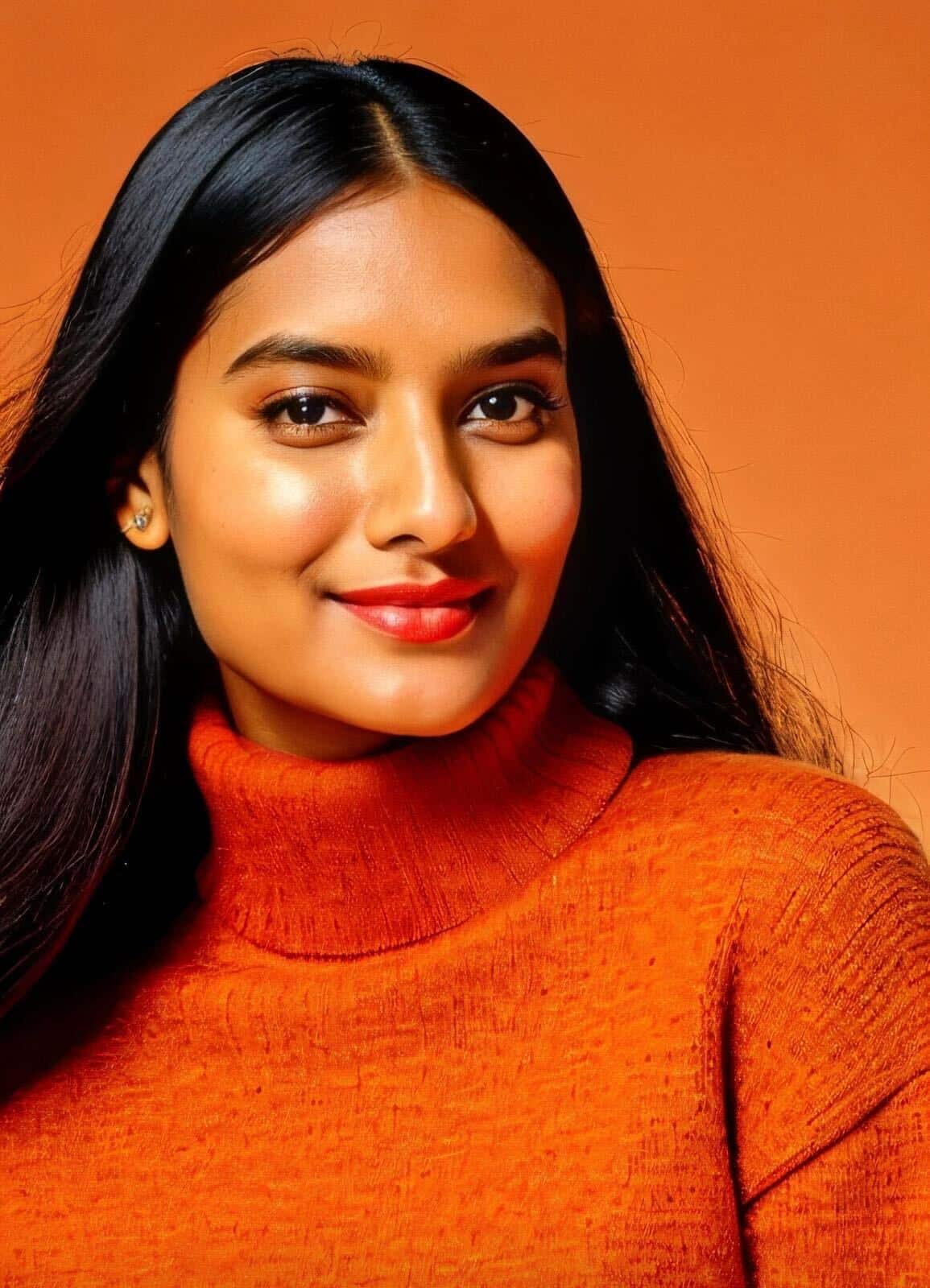
Sushma Kannedari
In this article, we will examine test cases for both IOS and Android platforms. Even if you are not currently testing on a specific platform, it’s beneficial to review both sections. Each example showcases different scenarios and features, enriching your understanding of Appium’s capabilities.
Let’s make our test case a little more sophisticated, while also looking at how we can work with iOS. If you are not planning on using iOS, we still suggest you read this section as we’ll be introducing new concepts applicable to both iOS and Android. For our iOS sample test case we will create a separate Test Case file named SampleIOS.java.
As we discussed above we need to put the iOS capabilities instead of Android capabilities, and define an IOSDriver class instead of an AndroidDriver class.
After specifying the desired capabilities, we can write the Automation test case. We have a sample app (.app file, which will work on iOS Simulator only) for automation. In this app, there is a feature where you can add 2 integer numbers and can get the results. So, we will automate this feature.
The steps to automate this would be:
1. Find the locator of TextField A and enter the value (ie. Send keys) from the keyboard.
driver.findElement(AppiumBy.id("IntegerA")).sendKeys(5 + "");
NOTE: The sendKeys() method accepts only String parameter, so we have converted the Integer value to a String by appending a blank String value.
2. Find the locator of TextField B and enter the second value from the keyboard.
driver.findElement(AppiumBy.id("IntegerB")).sendKeys(10 + "");
3. Find the locator of ‘Compute Sum’ and click on it, so the result would be displayed below the ‘Compute Sum’ textview.
driver.findElement(AppiumBy.id("ComputeSumButton")).click();
String answer = driver.findElement(AppiumBy.id("Answer")).getText();
NOTE: The getText() method is used to get the Text(in String format) from UI Elements.
4. Get the text of the result and compare it with the expected result, so if you enter 5 into TextField A, 10 into TextField B and when you click on ‘Compute Sum’ textview the result 15 should be displayed under ‘Compute Sum’.
Assert.assertEquals(answer, 15 + "", "Expected and Actual Result didn't match!");
NOTE: Assert.assertEquals(expected, actual, error_message) is a TestNG method used to compare the Expected and Actual values. This is the most important step of any test case, because this is how an automation test will know whether values being rendered on UI are correct and as expected or not. You will see us using Assertions throughout this guide.
TestNG is the Testing framework and works best with Appium (Mobile Automation) and Selenium (Website Automation), you can learn more about the TestNG Annotations and methods here.
5. Below is the full code of our test which will enter 2 values into text fields. Click on ‘Compute Result’, get the result from the app, and compare it with the expected result.
package com.example.appium;
import io.appium.java_client.AppiumBy;
import io.appium.java_client.ios.IOSDriver;
import io.appium.java_client.ios.options.XCUITestOptions;
import org.testng.Assert;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import java.net.MalformedURLException;
import java.net.URL;
public class SampleIOS {
public IOSDriver driver;
@BeforeTest
public void setUp() throws MalformedURLException {
// Set the desired capabilities for the iOS simulator
XCUITestOptions options = new XCUITestOptions();
options.setPlatformName("iOS");
options.setAutomationName("XCUITest"); // Use XCUITest for iOS automation
options.setDeviceName("iPhone 16 Plus"); // Change this if you're using a different simulator
options.setPlatformVersion("18.0"); // Your iOS version
options.setApp("/Users/sushmakannedari/Library/Developer/Xcode/DerivedData/TestApp-drwfzxxnlrpirqhhppcktmmkzdgy/Build/Products/Debug-iphonesimulator/TestApp.app");
options.setNoReset(true); // Prevents resetting the app state between sessions
// Appium server URL
URL appiumServerURL = new URL("http://127.0.0.1:4723"); // Update the URL if Appium runs elsewhere
// Initialize the iOSDriver
driver = new IOSDriver(appiumServerURL, options);
}
@Test
public void computeSumTest() {
// Step 1: Enter value in TextField A using Appium 2.0 syntax
driver.findElement(AppiumBy.id("IntegerA")).sendKeys(5 + "");
// Step 2: Enter value in TextField B using Appium 2.0 syntax
driver.findElement(AppiumBy.id("IntegerB")).sendKeys(10 + "");
// Step 3: Click on 'Compute Sum' using Appium 2.0 syntax
driver.findElement(AppiumBy.id("ComputeSumButton")).click();
// Step 4: Get the result and compare with expected value using Appium 2.0 syntax
String answer = driver.findElement(AppiumBy.id("Answer")).getText();
Assert.assertEquals(answer, 15 + "", "Expected and Actual Result didn't match!");
// Print success message
System.out.println("Test completed successfully. The computed sum is: " + answer);
}
}
You can get this example code on our github page.
After setting the valid Desired Capabilities, the next step is to pass them to the AndroidDriver class along with the Appium server URL (by default, it is http://127.0.0.1:4723/wd/hub).
The AndroidDriver is the primary class you will work with in your tests. Here’s how to set it up:
Here’s a code snippet demonstrating how to set this up:
Now let’s create the first sample Appium Test Case.
So let’s automate a simple scenario. In the below screen we want to click(tap) on the App Screen item from the list.
After writing the test case, we need to add the Appium logic to interact with the UI elements. In Appium, each element’s locator is essential for interaction. For example, if you want to tap on a button, you first need to find the locator of that button and then perform a `click()` action on it. We will explore locators in detail in a subsequent chapter.
For a deeper understanding of how to locate elements in Appium, you can refer to our blog on Appium Element Locator Strategies. This resource will provide you with various strategies and techniques for identifying UI elements effectively.
This code will find the Login Screen textview locator and simply click on it:
driver.findElement(AppiumBy.accessibilityId("App"));
appScreen.click();
Now our First Appium Automation Script is ready to execute, below is the complete code:
package com.example.appium;
import io.appium.java_client.AppiumBy;
import io.appium.java_client.android.AndroidDriver;
import io.appium.java_client.android.options.UiAutomator2Options;
import org.openqa.selenium.WebElement;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import java.net.MalformedURLException;
import java.net.URL;
public class SampleTest {
public AndroidDriver driver;
@BeforeTest
public void setUp() throws MalformedURLException {
// Initialize UiAutomator2Options
UiAutomator2Options options = new UiAutomator2Options();
// Set the desired capabilities for the Api Demos app
options.setPlatformName("Android");
options.setDeviceName("9B151FFAZ004ZQ"); // Replace with your emulator/device name
// Api Demos app package and activity
options.setAppPackage("io.appium.android.apis"); // Package name of the app
options.setAppActivity("io.appium.android.apis.ApiDemos"); // Main activity of the app
// Initialize the AndroidDriver with the Appium server URL and options
String appiumServerURL = "http://127.0.0.1:4723";
driver = new AndroidDriver(new URL(appiumServerURL), options);
}
@Test
public void openAppTest() {
// Locate the "App" element using Accessibility ID and click it
WebElement appScreen = driver.findElement(AppiumBy.accessibilityId("App"));
// Click on the "App" element
appScreen.click();
System.out.println("Clicked on the App screen successfully!");
}
}
Now you are ready to execute your test on a real device, so follow these steps:
Launch your terminal and type “appium” and Start the Server.
Connect your Android Mobile device to your computer and check that it is connected properly by executing the $ adb devices command. And also check the deviceName capability has the same name of the device which is showing up in the terminal.
Please make sure that device screen is unlocked and that it’s connected properly. Now, move to intelliJ Idea and select the test case name > Right click on it > Run ‘firstTest()’
Observe the Test Result and confirm the navigation on your device. It was a simple test case but you’ve actually accomplished a lot! From here, you get to explore all the cool features that Appium offers.
Congratulations on successfully completing your first Appium test case on a real device. This concludes our three part series on writing your first Appium test case. Along the way you learned a little bit about desired capabilities, locators and assertions. All of this is a great grounding to continue your education into the world of Automated testing and Appium.
Check out the rest of our Appium Test Case series:
Your First Appium Test Case: Setting up the IDE
Your First Appium Test Case: Writing and Running
To learn more, download our free eBook, Make the Move to Automation With Appium.