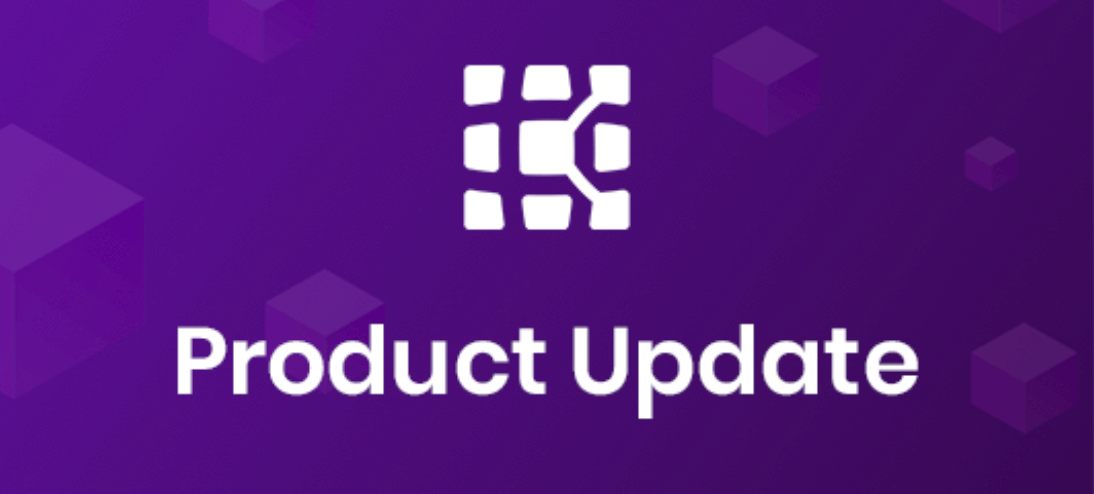
April 2021 Product Update: Better ways to create Appium scripts & more
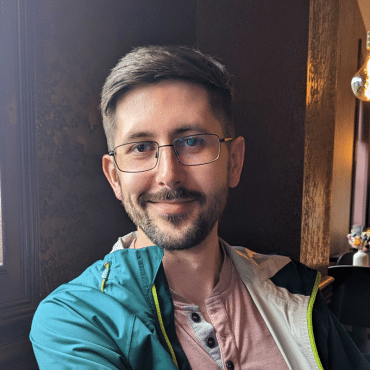
Adam Creamer
Everyone has a few daily tasks that can be easily handled by AI-assisted technology. Whether you’re browsing shows on a streaming device or in the testing and development phase of a current project, there’s a good chance that AI is standing by to help — if it hasn’t already.
You may already know how to approach the application testing process with some AI-powered testing tools. These can help you save time, achieve more dependable testing results, and even accelerate the software testing due timeline.
Regardless of your familiarity with AI as a testing tool, there’s a lot to learn. Today, we’ll share some ways that automation scripts can help you complete thorough, automated testing scripts and processes more efficiently.
What would you do with a few extra hours each day? There’s a good chance that there’s plenty on your to-do list that would benefit from a freed-up schedule.
Automation allows us to hand off business-critical tasks to AI tools, which allows you to focus on tasks that demand your active thought and engagement.
Automated test scripts are just another way that AI-powered automation is impacting our world today. Whether you’re just getting started with automated script testing – or if you’re an experienced pro – there’s always a lot to learn about the world of automated test scripts.
First, let’s clarify what automation scripts actually are. These targeted bits of code include testing launch and test data, and they are built with several variables connecting different values by which you can conduct testing processes in an automated fashion.
Various automation scripts exist today, and each one presents advantages to improve unique perspectives of the application testing process. Here are some of the most common:
Batch scripts store various commands which can be executed automatically, without so much as a single user intervention. Common applications might be loading programs, repetitive sequence actions, or when testing strategies require the operation of multiple processes.
Shell scripts are commands within a sequence targeting UNIX-based operating systems that are contained in a text file’s shell script.
When conducting cross-platform task automation, Powershell shines as a clear solution: it’s made up of a command-line shell, user interface, and a configuration management framework. Additionally, PowerShell works on Windows, Linux, and macOS.
When we consider commands within a file and programming language that has itself been designed to work like a program, then we’re talking about a Python script.
As you might imagine, a lot goes into automated scripting scenarios. That’s a good thing! It means that this tool is able to do even more than a first glance might suggest.
Components of an automation test script include input values, test steps, and expected outcomes. Let’s take a closer look at some of the other components that enable automation scripting to do its work.
The starting point of an automation or script code that triggers its execution.
Data placeholders within the script that store values and can be dynamically updated during runtime.
Instructions within the script that dictate actions to be performed.
Functions are script elements that manipulate data and provide return codes, and they’re distinct from regular script statements.
Any mechanism designed to manage errors and exceptions during script execution is referred to as error handling.
Okay, so you now understand just how great automation scripts can be, and some of the components that make it work. Ready to learn how to write an automation script, one step at a time? You’re in luck. Here we go!
Clearly identify the tasks or processes that you want to automate in test reports and determine the objectives and goals of automation. This will vary, and ultimately reflects part of your unique testing strategy.
Once you’ve defined the scope of your automation project, create test cases to validate the functionality and behavior of the automation by creating test scripts. These test cases can provide insight into additional test cases for automation.
Select the appropriate tools and technologies for developing and executing your automation scripts based on the requirements and constraints of your project. Kobiton’s AI-powered Appium script generator is just one helpful tool that can provide a major lift for your testing strategy.
Define the overall organization, modules, functions, classes, and dependencies of your scripts. Again, this will depend upon your goals and objectives, which are unique to the product in development.
Break down the tasks or processes that you want to automate into smaller, manageable units. This is a great piece of advice for any project, and it applies to software application testing as well.
Once you’ve broken down the tasks, determine the sequence of actions or steps that your automation scripts need to perform. Be sure that expectations are uniform across your team, and that everyone properly anticipates the automation steps that will be taking place.
Implement robust error handling mechanisms to detect, handle, and recover from errors and exceptions in your automation scripts. Tools like these can save time, and – ultimately – team resources.
Before deploying your own automation frameworks and scripts in a production environment, thoroughly test them to verify their functionality, correctness, and reliability.
Once your automation scripts have been tested and validated, deploy them in your production environment to automate the intended tasks or processes. AI-powered resources are continually improving the testing process, and a greater familiarity with them can improve nearly any project.
Alright — you’re feeling pretty good about automation scripts by now, and you’re ready to step up your knowledge to include some advanced concepts. We’ve got you covered.
These are techniques to handle dynamic elements on web pages that may change based on user interactions or data updates. Due to the nature of dynamic elements, it’s difficult to summarize every possible scenario here; that’s where expert advisors at Kobiton are able to help out.
When we conduct parallel test execution, we run multiple automation scripts simultaneously. Doing so allows us to speed up testing processes and improve efficiency, which are cornerstones of the automated testing landscape.
Cross-browser testing is the process of writing scripts that can execute tests across different web browsers to ensure consistent functionality and user experience. End users will likely work with the software in development across numerous devices, and you’ll want to conduct cross platform testing to prepare for those scenarios.
This is integrating the automated scripting with Continuous Integration/Continuous Deployment (CI/CD) pipelines for automated testing in the software development lifecycle.
# Automation Script to Modify Media
def modify_media(media_file):
# Code to open and modify the media file
print(f”Modifying media file: {media_file}”)
# Additional code for modifying media content
print(“Media modification completed successfully”)
# Usage
modify_media(“example.mp4”)
import pandas as pd
# Automation Script to Read a CSV
def read_csv(csv_file):
# Code to read and process the CSV file
data = pd.read_csv(csv_file)
print(“CSV file contents:”)
print(data)
# Usage
read_csv(“data.csv”)
# Automation Script to Import Live Data
def import_live_data(source):
# Code to import live data from a specified source
print(f”Importing live data from: {source}”)
# Additional code for processing live data
print(“Live data import completed successfully”)
# Usage
import_live_data(“https://example.com/live-data”)
import pygame
# Automation Script to Play Music
def play_music(music_file):
# Code to play music from a specified file
pygame.init()
pygame.mixer.music.load(music_file)
pygame.mixer.music.play()
# Usage
play_music(“song.mp3”)
import pyttsx3
# Automation Script for Automatic Text to Speech
def text_to_speech(text):
# Code to convert text to speech automatically
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
# Usage
text_to_speech(“Hello, welcome to the automated text-to-speech script”)
Like every tool and concept, there’s a lot of value in getting the most out of it. Fortunately, an investment into ongoing education about growing trends and AI capabilities can make all the difference.
So – without further ado – here are some of my personal favorite tips and tricks for better test automation frameworks and scripting. These points can ensure that you write test scripts that are efficient, reliable, maintainable, and scalable.
Use try-catch blocks, exception-handling routines, logging, and reporting to ensure script robustness and reliability.
I like to tell clients it’s important to avoid complexity. Keep it simple! Promote code efficiency by implementing reusable functions and modules in your automation scripts.
Integrate test automation into the development process to create more automated tests in a zero-bug environment and save time. Resources are often in limited supply, and test automation tools can turn your casual stroll into a sprint.
Combine manual testing with automation testing for optimal test results, ensuring comprehensive test coverage. Some advantages accompany each, and the most effective testing strategies are in-depth and thorough.
It’s no secret that AI-powered technology and solutions are changing the world we live in, and you don’t have to work in tech to understand the impact it has on how we all get things done. At Kobiton, we understand the ways that new solutions can affect our productivity and can strengthen our competitive edge.
Scriptless test automation is just one of many AI solutions at our disposal during the mobile application testing and development process.
Automated automation test scripts that can help you work smarter — by saving time, acquiring more dependable results, and zipping toward project deadlines.
For those looking to dive deeper into the nuances of mobile testing and stay ahead of emerging trends, joining Kobiton at the MTES 2024 on May 16, offers a perfect opportunity. It’s an invitation to connect with industry leaders, gain invaluable insights, and explore innovative solutions that drive mobile excellence. Don’t miss out, register now for MTES 2024 and transform your testing strategy.